The article discusses the importance of checking for null values in programming to prevent Null Pointer Exceptions, ensure data integrity, and handle empty states. It emphasizes the role of null checks in preventing crashes, validating input, improving user experience, defining clear business logic, and enhancing code quality. The article also provides specific examples and best practices for null checks in Java, such as using `Objects.requireNonNull()` and `StrUtil.isNotBlank()`. Overall, the article underscores the significance of null checks in software development to create robust and reliable applications.
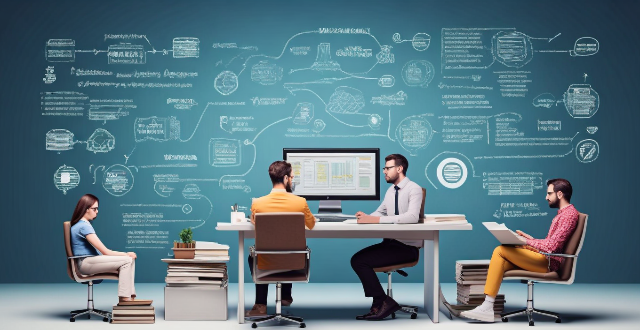
Checking for null is crucial in coding to prevent Null Pointer Exceptions, ensure data integrity, and handle empty states. In programming, null represents an uninitialized or absent value. Failing to check for null can lead to unexpected errors, particularly Null Pointer Exceptions (NPE), which occur when an application tries to invoke methods or access variables on a null object. Here's a detailed exploration of the significance of checking for null:
1. Avoiding Null Pointer Exceptions
- Crash Prevention: An unhandled Null Pointer Exception can cause the program to crash, leading to a poor user experience and system instability.
- Type Differences: In languages like Java, checking for null is essential because different data types require different null checks. Strings and Integers, for example, have distinct ways to check for null values.
2. Ensuring Data Integrity
- Validation and Security: Checking for null helps maintain data integrity by ensuring that only valid data is processed, which is critical for security and data consistency.
- Input Validation: Proper null checks can prevent injection attacks where malicious input could exploit unused or uninitialized variables.
3. Handling Empty States
- User Experience: Correctly handling null values leads to better user interface design, providing clear feedback when no data is available or when an operation fails due to missing input.
- Logical Operations: When working with lists or streams, understanding the concept of "vacuous truth" (a logical statement being true by default if there are no elements to contradict it) is vital for correctly implementing logic that involves empty collections.
4. Defining Clear Business Logic
- Unambiguous Interfaces: Using tools like Java 8's
Optional
for returning values can help clearly define the possibility of absent values, making the business logic more explicit and reducing confusion. - Preventing Bugs: A significant number of bugs can be prevented by thoroughly checking for null values, especially during initial development stages. This practice can save debugging time and resources.
5. Improving Code Quality
- Documentation and Readability: Proper documentation and use of tools that help indicate nullable vs. non-nullable types (e.g., using
@Nullable
annotations) make the code more readable and easier to maintain. - Design Patterns and Best Practices: Applying design patterns and best practices related to null checks contributes to the overall quality and robustness of the codebase.
Additionally, it's important to understand the nuances of null checks within the specific programming language and environment you are working with. For instance, in Java:
- Using
Objects.requireNonNull()
can help in validating inputs at the beginning of a method, ensuring that null values do not propagate through the application. - Being aware of methods like
StrUtil.isNotBlank()
for strings, which checks both for null and whitespace, can prevent certain categories of errors right from string processing functions.
In conclusion, checking for null is a defensive programming strategy that helps developers anticipate and handle absence of value gracefully, which in turn enhances the reliability and user satisfaction of software applications.