JavaScript handles `null` and `undefined` in a specific way. `null` is an assignment value that represents no value or no object, and it is considered falsy in boolean contexts. On the other hand, `undefined` is a type of variable that has been declared but not assigned a value, also considered falsy in boolean contexts. The difference between them lies in their intention: `null` indicates an explicit assignment indicating no meaningful value, while `undefined` means the variable has been declared but not yet assigned a value. To check if a variable is `null` or `undefined`, you can use the strict equality (`===`) operator. Understanding these concepts allows developers to write more robust code that manages variable states appropriately.
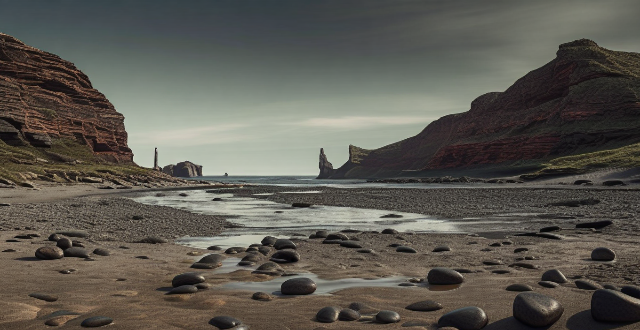
JavaScript handles null
and undefined
in a specific way.
Null
null
is an assignment value that represents no value or no object. It is considered falsy in boolean contexts, meaning it will evaluate to false
when used in conditions like if
, while
, etc.
Example:
let myVar = null;
console.log(myVar); // Output: null
console.log(Boolean(myVar)); // Output: false
Undefined
undefined
is a type of variable that has been declared but not assigned a value. It is also considered falsy in boolean contexts.
Example:
let myVar;
console.log(myVar); // Output: undefined
console.log(Boolean(myVar)); // Output: false
Difference between null and undefined
null
is an intentional assignment to a variable indicating that the variable does not hold any meaningful value.undefined
means that a variable has been declared but has not yet been assigned a value.
Example:
let myVar1 = null; // The variable has been assigned the value null explicitly.
let myVar2; // The variable has not been assigned any value, so its default value is undefined.
Checking for null or undefined
To check if a variable is null
or undefined
, you can use the strict equality (===
) operator.
Example:
let myVar1 = null;
let myVar2;
if (myVar1 === null || myVar1 === undefined) {
console.log("myVar1 is either null or undefined");
} else {
console.log("myVar1 has a value");
}
if (myVar2 === null || myVar2 === undefined) {
console.log("myVar2 is either null or undefined");
} else {
console.log("myVar2 has a value");
}
In conclusion, JavaScript provides clear distinctions between null
and undefined
to help developers manage variables more effectively. By understanding these concepts, you can write more robust code that handles variable states appropriately.